Plan B
When we decided we wanted to implement our own graphics module we first investigated how to draw sprites to the screen. There are two main ways to draw sprites - via hardware or by dynamically drawing sprites to the video memory (also known as bit blitting).
Bit blitting, which we did not investigate in detail, is different from hardware sprites in the way it draws to the screen. Bit blitting draws directly to the video memory that the rest of the display draws to (typically the background). Because of this, it has the advantage of being able to draw any bitmap to the screen, allowing game creators to dynamically create bitmaps and draw the results to the screen.
However, back in the days of Pac-Man, processor speed and memory capacity were both extremely low, rendering bit blitting nearly impossible. The original Pac-Man CPU was clocked at 3.072 MHz and only used a total of 20K of memory. This memory not only included the video memory that was drawn to the screen, but all memory available to the programmers to load and store data. Because of these restrictions, dynamically creating and drawing bitmaps to the screen was impossible.
Hardware Sprites
Hardware sprites differ from bit blitting in that sprites are hard coded into the hardware of the system. In Pac-Man, such sprites would be Pac-Man himself, the ghosts, the dots, and the fruit. The backgrounds are static images stored in a different set of memory. The hardware can call the sprite module to draw any predefined sprite to the video memory. This eliminates the burden on the processor to draw to the video memory, resulting in faster performance. Furthermore, since the hardware never creates sprites dynamically and instead updates the video memory with predefined sprites, the entire system requires less memory on the whole.
Our Hardware Sprite Module
The original sprite module in Pac-Man can fetch any predefined sprite from memory, color it, and display it in any horizontal or vertical orientation. We initially tried to emulate this, but due to time constraints opted to simplify the module.
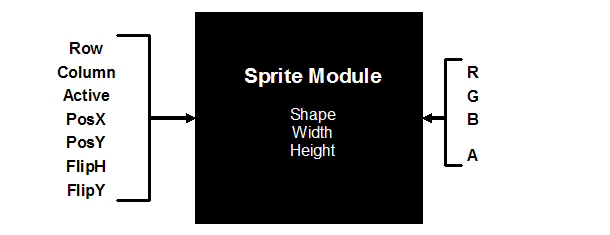
The above diagram shows how our module is designed. Position and orientation parameters are sent to the module, within which the shape, size, and color of the sprite are defined. Output to the VGA port is in the form of Red, Green, Blue, and Active values.
Our first iteration of the sprite module simply drew a 16x32 blue rectangle anywhere on the screen. In reality, this module removed the ability to fetch sprites and always drew a blue rectangle since it was hard coded into the module. This module works by sending a "1" to the blue output pin of the VGA port on the FPGA as long as the current row and column being drawn by the VGA module are both within the boundary of our rectangle. We also added the ability to move the rectangle around the screen via the buttons on the FPGA. This simply incremented or decremented the horizontal and vertical positions of the rectangle whenever the input signal from the buttons was high.